Can we iterate in unordered_map C++?
Here’s a simple explanation:
unordered_map in C++ is a powerful data structure that implements hash tables. This means that the elements are stored in a way that allows for extremely fast access based on their keys. While unordered_maps are great for quick lookups, sometimes we need to process every single element within them. This is where iterators come in.
Iterators are like pointers that allow us to traverse through the data stored in a container. Think of them as guiding hands that help you navigate through the unordered_map. They offer a way to visit each key-value pair stored within.
Let’s look at a code example:
“`c++
#include
#include
int main() {
std::unordered_map
{“Apple”, 1},
{“Banana”, 2},
{“Cherry”, 3}
};
// Iterating over the unordered_map
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
return 0;
}
```
This code snippet creates a unordered_map named myMap and populates it with some fruit names and their corresponding values. Then, it uses a for loop and an iterator named it to step through each key-value pair in the unordered_map. Within the loop, we print the current key (it->first) and its corresponding value (it->second).
Let’s break down what’s happening in the code:
1. `myMap.begin()`: This returns an iterator pointing to the first element in the unordered_map.
2. `myMap.end()`: This returns an iterator that points past the last element in the unordered_map.
3. `it != myMap.end()`: This condition checks if the current iterator it has reached the end of the unordered_map. If not, the loop continues.
4. `++it`: This moves the iterator it to the next element in the unordered_map.
5. `it->first`: This accesses the key of the current element pointed to by it.
6. `it->second`: This accesses the value associated with the key of the current element pointed to by it.
Using iterators allows us to work with each element in the unordered_map one by one, making it possible to perform various operations, such as:
– Modifying values: You can update the value associated with a key by modifying it->second.
– Deleting elements: You can remove an element by using myMap.erase(it), which erases the element pointed to by it.
– Searching for specific keys: You can search for a specific key by iterating through the unordered_map and comparing the key with it->first.
In short, iterators empower you to work with the elements of an unordered_map in a sequential manner, allowing you to perform many useful operations.
What is an iterator in unordered_map?
Think of an iterator as a pointer that helps you navigate your way through the items stored in an unordered_map. Just like a pointer, it can point to the current element you’re looking at.
The `begin()` method gives you an iterator that points to the first element in the unordered_map. If the unordered_map is empty, the `begin()` method returns a special iterator called the past-the-end iterator. This iterator is like a placeholder that indicates the end of the container. It doesn’t actually point to any element.
Iterators are really helpful for many reasons. For example, you can use them to:
Access elements: You can use an iterator to retrieve the data associated with a key in the unordered_map.
Loop through the map: You can loop through the elements in an unordered_map using an iterator.
Modify elements: You can use an iterator to change the values associated with keys in the unordered_map.
Here’s a simple example to illustrate:
“`c++
#include
#include
int main() {
// Create an unordered_map
std::unordered_map
// Get an iterator to the beginning of the map
std::unordered_map
// Loop through the map and print the key-value pairs
for (; it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
return 0;
}
```
This code would print the following output:
```
cherry: 3
banana: 2
apple: 1
```
As you can see, the loop iterates through each key-value pair in the unordered_map, using the iterator to access the elements.
Understanding iterators is essential for working efficiently with unordered_maps. They provide a powerful mechanism to manipulate and traverse data stored in these containers.
What is the unordered_map dictionary in C++?
Let’s break down how it differs from a regular map. The map in C++ is also a dictionary, but it keeps its keys in a sorted order. This means that map operations like inserting or searching can be slightly slower, but it guarantees that the keys are always sorted. Unordered_map doesn’t bother with sorting, so it can be much faster, especially for larger datasets.
Here’s a simple analogy: imagine you have a box of tools. If the tools are arranged in a specific order (like a map), you might have to look around a bit to find the right one. But if the tools are just thrown in randomly (like an unordered_map), you might find the tool you’re looking for more quickly.
Unordered_map uses a hash table to store its data. This means that each key is converted into a number called a hash value, and this value is used to determine the location where the key-value pair is stored. This allows for incredibly fast lookups, insertions, and deletions of key-value pairs.
Here’s a simple example of how you might use an unordered_map in C++:
“`c++
#include
#include
int main() {
// Create an unordered_map to store student names and their corresponding grades
std::unordered_map
// Add some students and their grades
studentGrades[“Alice”] = 95;
studentGrades[“Bob”] = 88;
studentGrades[“Charlie”] = 75;
// Access a student’s grade
std::cout << "Alice's grade: " << studentGrades["Alice"] << std::endl;
// Check if a student exists
if (studentGrades.count("David") > 0) {
std::cout << "David's grade is in the map." << std::endl;
} else {
std::cout << "David's grade is not in the map." << std::endl;
}
return 0;
}
```
This example shows how you can add, access, and check for the existence of key-value pairs in an unordered_map. It's a versatile data structure that can be used in a wide variety of applications, such as storing and retrieving data from databases, implementing caches, and building efficient search algorithms.
What is the difference between map and unordered_map?
map stores elements in ascending order based on their keys. This means that if you insert elements into a map, they will be automatically arranged in sorted order, making it easy to iterate through them in a predictable sequence.
unordered_map, on the other hand, doesn’t maintain any specific order for its elements. It uses a hash table to store the key-value pairs, which allows for fast access and insertion operations. The order of elements in an unordered_map is not guaranteed and can change over time.
Let’s visualize this with an example:
Imagine you have a collection of students and their corresponding grades.
If you use a map to store this data, the students will be arranged alphabetically based on their names. If you iterate through the map, you’ll get the students in alphabetical order, starting from ‘A’ and going to ‘Z’.
However, if you use an unordered_map, the students will be stored in no particular order. When you iterate through it, the order might be random or might change after inserting or deleting elements.
Here’s a summary of the key differences:
| Feature | map | unordered_map |
|—————–|—————————————————|——————————————|
| Order | Sorted by key (ascending order) | No specific order |
| Implementation | Binary search tree | Hash table |
| Access Time | O(log n) (average) for insertion, deletion, and access | O(1) (average) for insertion, deletion, and access |
| Memory Usage | More memory overhead than unordered_map | Less memory overhead than map |
Choosing the right container:
– If you need to iterate through the elements in a specific order, use map.
– If you need fast access and insertion operations, use unordered_map.
– If memory usage is a concern, use unordered_map.
Keep in mind that the choice between map and unordered_map depends on your specific needs and priorities.
How to iterate over a map of maps in C++?
This technique provides a clean and efficient way to access and process each element within your nested map structure.
The std::for_each algorithm acts as a versatile tool for applying a specific function to each element in a range.
We can leverage this by using a lambda function as the call-back function.
This lambda function will be executed for each map entry, giving you the freedom to perform any operation you need on those entries.
Here’s how it works in practice:
Imagine you have a map of maps, where the outer map holds strings as keys, and the inner maps also use strings as keys. Each inner map stores integers as values.
“`c++
#include
#include
How to use unordered_map in C?
This is how unordered_map works. Imagine you have a collection of student names and their respective grades. You could use an unordered_map to store this information. Each student’s name would be a key, and their grade would be the value associated with that key.
The unordered_map is incredibly useful for keeping track of data when you need to look up values based on unique identifiers. In our student example, if you wanted to know John’s grade, you would use his name (the key) to access his grade (the value).
But how do you actually use an unordered_map in C++? Let’s break it down:
1. Include the header file: You’ll need to include the `
2. Declare the map: You’ll declare a unordered_map object and specify the data types for your keys and values. For example:
“`c++
std::unordered_map
“`
This line creates an unordered_map called studentGrades, where the keys are std::string (representing student names) and the values are int (representing their grades).
3. Insert elements: You can add elements (key-value pairs) to the unordered_map using the `insert()` method. For example:
“`c++
studentGrades.insert({“John”, 85});
studentGrades.insert({“Jane”, 92});
“`
4. Access elements: To access the value associated with a specific key, use the `[]` operator. For example:
“`c++
int johnsGrade = studentGrades[“John”];
“`
This line retrieves John’s grade, which is stored in the `johnsGrade` variable.
5. Iterate through the map: You can loop through the elements of the unordered_map using iterators. This allows you to process each key-value pair in the map.
“`c++
for (auto it = studentGrades.begin(); it != studentGrades.end(); ++it) {
std::cout << it->first << " : " << it->second << std::endl;
}
```
This loop will print out the names and grades of all the students stored in the `studentGrades` map.
So, there you have it! That's the basic rundown of how to use unordered_map in C++. It's a powerful tool for organizing and accessing data efficiently. The key takeaway is that an unordered_map is all about associating keys with their corresponding values, making it a fantastic choice for creating data structures that need to be accessed quickly and easily.
Which is faster, map or unordered_map?
std::unordered_map uses a hash table to store its key-value pairs. This means that the insertion process involves hashing the key and then directly accessing the corresponding bucket in the hash table. If the bucket is empty, the key-value pair is simply inserted. If the bucket already contains other elements, the map will need to perform a linear search within that bucket to find the correct place for the new element. However, even with a linear search within a bucket, the time complexity of insertion in a hash table remains constant on average.
std::map, on the other hand, utilizes a balanced binary search tree (often a red-black tree) to organize its elements. Insertion in a binary search tree involves traversing the tree from the root node down to the appropriate location where the new node should be placed. The time complexity of this traversal is logarithmic, as it depends on the height of the tree. This means that as the size of the map grows, the time required for insertion increases logarithmically.
In summary, the advantage of std::unordered_map stems from its use of a hash table, which provides constant average insertion times, making it a faster choice for scenarios where frequent insertions are required. std::map, while offering efficient searching and ordered traversal, comes at the cost of slower insertion operations due to its reliance on a balanced binary search tree.
See more here: What Is An Iterator In Unordered_Map? | How To Iterate Unordered_Map C++
See more new information: bmxracingthailand.com
How To Iterate Unordered_Map C++ | Can We Iterate In Unordered_Map C++?
Hey there, C++ programmers! Today, we’re diving into the exciting world of iterating over unordered maps. So, if you’re looking to navigate the ins and outs of this powerful data structure, you’ve come to the right place. Let’s break it down together.
The Power of Unordered Maps
Unordered maps, our star players in C++, are a form of associative containers that store elements in key-value pairs. The magic lies in the fact that they use hash tables to ensure quick access to data. Think of it as a super-efficient way to store and retrieve information – just like a lightning-fast search engine.
Iteration Basics: The Essential Tools
When it comes to iterating over an unordered map, there are two main approaches, both employing the power of iterators:
The Classic `begin()` and `end()` Duo: This is the classic way to traverse a container. `begin()` points to the first element, and `end()` points to the imaginary element after the last one. We use a `for` loop to move through the map, element by element.
The Range-Based `for` Loop: This is a modern and convenient approach, especially for straightforward iteration. It automatically handles the iterators for us, making the code a bit cleaner.
Code Time: Putting It Into Practice
Let’s get our hands dirty with some code examples. We’ll create a sample unordered map and then demonstrate the two iteration methods:
“`c++
#include
#include
#include
int main() {
// Creating a sample unordered map
std::unordered_map
{“Apple”, 1},
{“Banana”, 2},
{“Cherry”, 3},
{“Dates”, 4}
};
// Iteration Method 1: Using `begin()` and `end()`
std::cout << "Using begin() and end():\n";
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
// Iteration Method 2: Using Range-Based `for` Loop
std::cout << "\nUsing Range-Based for Loop:\n";
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
```
Let's break down the code:
1. We include the necessary headers `
2. We create a sample `unordered_map` named `myMap`. It’s populated with key-value pairs where the keys are strings and the values are integers.
3. Method 1: We use a `for` loop with `begin()` and `end()`. The iterator `it` is initially set to `myMap.begin()`. The loop continues as long as `it` is not equal to `myMap.end()`. Inside the loop, we print the key (`it->first`) and value (`it->second`) of the current element. We use `++it` to move to the next element.
4. Method 2: We use a range-based `for` loop, which is more concise. The loop automatically iterates over each element in `myMap`. We access the key and value using `pair.first` and `pair.second`, respectively.
Key Points to Remember
No Guaranteed Order: Unlike `map`, an `unordered_map` doesn’t provide any guarantee about the order in which elements are iterated. This is because the underlying hash table implementation doesn’t store elements in a sequential fashion.
Iterators and Modifying: Be cautious when modifying the unordered map during iteration. If you need to add or remove elements while iterating, use `unordered_map.insert()` and `unordered_map.erase()` carefully. It’s generally best to avoid modifications during iteration to prevent unexpected behavior.
FAQs: Solving Your Unordered Map Iteration Questions
1. How can I iterate over the keys of an unordered map?
* You can access the keys directly using the iterator’s `first` member. For example:
“`c++
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << std::endl; // Prints only the keys
}
```
2. How can I iterate over the values of an unordered map?
* You can access the values directly using the iterator's `second` member. For example:
```c++
for (const auto& pair : myMap) {
std::cout << pair.second << std::endl; // Prints only the values
}
```
3. What are the differences between iterators for `map` and `unordered_map`?
* While both `map` and `unordered_map` use iterators for traversal, `map` iterators maintain the order of the elements based on the keys. In contrast, `unordered_map` iterators don't guarantee any specific order, making iteration behavior more unpredictable.
4. Can I use `for_each` to iterate over an unordered map?
* You can use `for_each` with a custom function to iterate over an unordered map, but it's not as common as using `begin()` and `end()` or the range-based `for` loop. `for_each` can be useful when you want to apply a function to each element of the map.
Wrapping It Up
And there you have it! We've explored the key ways to iterate over `unordered_map` in C++, including the `begin()` and `end()` methods, the range-based `for` loop, and a peek into how to access keys and values during iteration. Remember, mastering iteration is a vital skill for unlocking the power of C++'s unordered map data structure. Happy coding!
Traversing a Map and unordered_map in C++ STL – GeeksforGeeks
We can traverse map and unordered_map using 4 different ways which are as follows: Using a ranged based for loop. Using begin () and end () Using Iterators. Using std::for_each and lambda function. 1. Using a Range Based for Loop. We can use a GeeksForGeeks
c++11 – Iterating over unordered_map C++ – Stack Overflow
If you prefer to keep intermediate data in sorted order, use std::map
How to iterate over an unordered_map in C++11 – thisPointer
In this article we will discuss the different ways to iterate over an unordered_map. First lets create an unordered_map and then we will see the different thisPointer
std::unordered_map – cppreference.com
Two keys are considered equivalent if the map’s key equality predicate returns true when passed those keys. If two keys are equivalent, the hash function must CPP Reference
How to use unordered_map efficiently in C
The unordered_map::begin() is a built-in function in C++ STL which returns an iterator pointing to the first element in the unordered_map container or in any of its GeeksForGeeks
What Is Unordered_map in C++: Types, Iterators
Discover unordered_map in c++ by undersatnding what it means. Explore its public types, iterators, member types and methods of an unordered_map in C++. Simplilearn
unordered_map in C++ STL – GeeksforGeeks
The unordered_map::end() is a built-in function in C++ STL which returns an iterator pointing to the position past the last element in the container in the unordered_map container. In an GeeksForGeeks
std:: unordered_map – The C++ Resources Network
Aliased as member type unordered_map::allocator_type. In the reference for the unordered_map member functions, these same names (Key, T, Hash, Pred and Alloc) C++ Forum
How to loop through elements in unordered map in C
The unordered map provides begin () and end () member functions which can be used to get iterators to the first element and the one after the last. The following code illustrates codespeedy.com
unordered_map – C++ Users
Returns an iterator pointing to the first element in the unordered_map container (1) or in one of its buckets (2). Notice that an unordered_map object makes no guarantees on cplusplus.com
Maps In C++ (Std::Map And Std::Unordered_Map)
Std::Unordered_Map In C++ | Stl C++
Iterate Map Keys And Values In C++
Iterate Over Map In C++11/17
#16 [C++]. Cấu Trúc Dữ Liệu Map Trong C++ | Multimap | Unordered_Map
Iterators In C++
Map Vs Unordered_Map In C++ | Stl Tutorials
Map Vs Unordered Map In C++
Link to this article: how to iterate unordered_map c++.
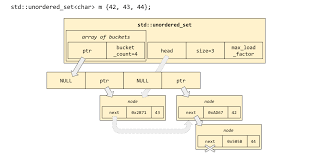
See more articles in the same category here: https://bmxracingthailand.com/what